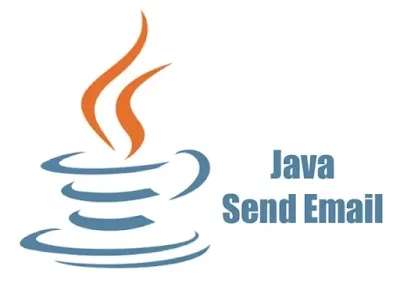
Introduction
One of the most common and necessary requirement for most applications is sending emails. The JavaMail API provides a platform-independent and protocol-independent framework for building mail and messaging applications.
The JavaMail API is available as an optional package for use with the Java SE platform and is also included in the Java EE platform. The JavaMail reference implementation is released under the GNU General Public License (GPL) v2 with Classpath Exception and the Common Development and Distribution License (CDDL) v1.1.
Getting Started
Through this guide let's discuss how to set up email sending feature in your Java project and use the JavaMail API to create and send emails using the SMTP protocol. So, let's create a spring boot project using maven as the dependency management tool.
1. Adding Dependencies
Here are the dependencies you need to add to your pom.xml in order to work with Java Mail Sender.
pom.xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
2. Configure Application Settings
In order to work with Java Mail Sender you also need to define these spring.mail property settings in the application.yml file as below.
application.ymlspring:
application:
name: email-application
...
mail:
host: <smtp-mail-server-host>
port: <smtp-server-server-port>
username: <mail-username>
password: <mail-password>
properties:
mail:
transport:
protocol: smtp
smtp:
auth: true
starttls:
enable: true # enable TLS
connectiontimeout: 6000
timeout: 6000
writetimeout: 6000
from: <sender-email-address>
3. Creating Email Component to Send Emails
Using Java Mail Sender, emails can be sent as several types with different options. It allows send HTML embedded mails, mails with plain text or plain text mails with attached documents etc.
EmailComponent.java
package com.devsdebugger.email.component;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.ByteArrayResource;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Component;
import javax.mail.MessagingException;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
@Component
@RequiredArgsConstructor
public class EmailComponent {
private final JavaMailSender mailSender;
@Value("${spring.mail.from}")
private String senderEmailAddress;
/**
* Generates and sends a HTML embedded email to provided recipients
*/
public void sendHtmlEmbeddedEmail(String htmlMsg, String recipientEmail,
String subject) throws MessagingException {
MimeMessage mimeMessage = mailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(mimeMessage, "UTF-8");
helper.setFrom(senderEmailAddress);
helper.setTo(recipientEmail);
helper.setSubject(subject);
helper.setText(htmlMsg, true);
mailSender.send(mimeMessage);
}
/**
* Generates and sends a plain text email with attachments to provided recipients
*/
public void sendEmailWithAttachments(String recipientEmail, String subject,
String emailText, String fileName,
byte[] workbookData) throws MessagingException {
MimeMessage message = mailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setFrom(new InternetAddress(senderEmailAddress));
helper.setTo(recipientEmail);
helper.setSubject(subject);
helper.setText(emailText);
helper.addAttachment(fileName, new ByteArrayResource(workbookData));
mailSender.send(message);
}
}
Now we're ready to work with Java Mail Sender and send emails to any of the given
recipients. You may use the below piece of code to invoke the particular methods
defined in the email component in order to send emails according to the expected
format either html embedded or plain text with attachments.
Using the HTML embedded format, you have the ability to format text such as making
them bold, italic or underlined and adding some images using Hypertext Markup
Language. Using Plain Text is regular text with no other special formatting options
such as bold, italics, underlines or other special layout options.
// Invokes HTML Embedded Email Send method in Email Component
emailComponent.sendHtmlEmbeddedEmail(htmlMsg.toString(), emailAddress,
String.format(smsStatusReportEmailSubject, displayReportingType));
// Invokes Plain Text Email with Attachments Send method in Email Component
emailComponent.sendEmailWithAttachments(emailAddress, subject, emailText,
subject.concat(".xlsx"), getWorkbookAttachment());
Keywords:
learn java
java basics
java
jdk
java jdk
spring framework
java programming
java development kit
java spring
java code
java programming language
java language
0 Comments